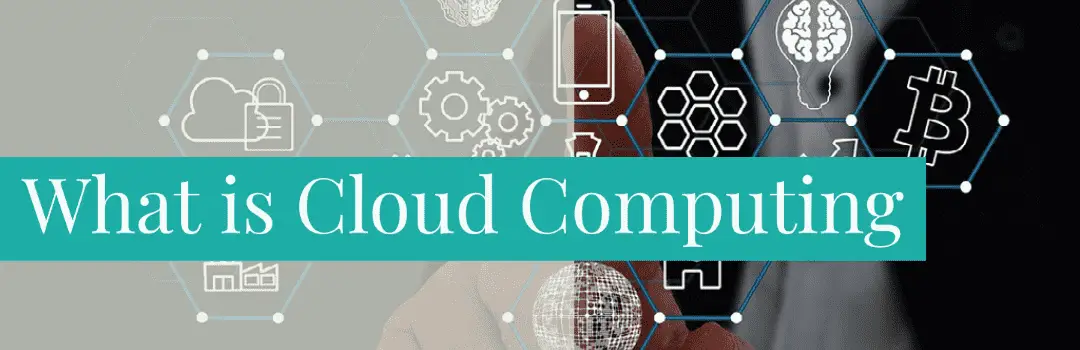
10
Apr
Confused whether to use PUT or PATCH or POST in your API? Don’t worry you are not alone and this is a common confusion which happens to most of us or to all of us at some point while developing our API. Before we understand the difference between PUT vs PATCH vs POST, first we need to understand Idempotency.
Idempotence is the property of certain operations in mathematics and computer science, that can be applied multiple times without changing the result beyond the initial application.
To put the above quote in terms of APIs
To simply put, idempotency can be described as an API call when done multiple times provides the same result. For example, the GET method requests a certain blog post. No matter how many times it is called the same blog post is returned (content can vary) in the same format. While the returned value can vary with updates to the post, the content format is always same.
HTTP request methods or ‘verbs’ equivalent to the CRUD, but they are not the same and serve different purpose
HTTP Method | Idempotent |
---|---|
OPTIONS | Yes |
GET | Yes |
HEAD | Yes |
PUT | Yes |
POST | No |
PATCH | No |
DELETE | Yes |
Now that we have an idea about idempotency, lets understand POST, PUT and PATCH and where does the confusion arise.
POST is a HTTP method used to create a new resource in a collection of resources. POST method should ideally be used only to create new resources. Since REST doesnt have a standard set of rules, some APIs use POST to update a resource as well. This is better to avoid. Use POST only to create a resource.
On successful creation, return HTTP status 201, returning a Location header with a link to the newly-created resource.
Lets look at this example: When you POST the following payload to the url
HTTP POST https://programmerspub.com/blog/general
Payload:
{
"blog_title": "What is POST",
"blog_post": "Confused whether to use PUT or PATCH or POST...",
"blog_post_author": "programmerspub"
}
We get this following response
{
"blog_post_id": 3
"blog_title": "What is POST",
"blog_post": "Confused whether to use PUT or PATCH or POST...",
"blog_post_author": "programmerspub"
}
If you do the same request 2 times, 2 resources are created. Due to this reason, POST methond is not idempotent and unsafe.
{
"blog_post_id": 4
"blog_title": "What is POST",
"blog_post": "Confused whether to use PUT or PATCH or POST...",
"blog_post_author": "programmerspub"
},
{
"blog_post_id": 3
"blog_title": "What is POST",
"blog_post": "Confused whether to use PUT or PATCH or POST...",
"blog_post_author": "programmerspub"
}
POST method is non-idempotent and is cacheable.
PUT is another HTTP method used to create a new resource at a specified URI or to update an existing resource. Although PUT can be used to create a resource, it is most often used to update resource. To create a new resource, using PUT we need to know the exact URI where the data needs to be put. If incase there is data in the specified URI, the entire data is overwritten which is update.
To create a resource at specified URI /5:
HTTP PUT https://programmerspub.com/blog/5
Payload:
{
"blog_title": "What is PUT",
"blog_post": "Confused whether to use PUT or PATCH or POST...",
"blog_post_author": "programmerspub"
}
To update a resource:
HTTP PUT https://programmerspub.com/blog/5
Payload:
{
"blog_title": "What is PUT",
"blog_post": "Confused whether to use PUT or PATCH or POST...updated post...",
"blog_post_author": "programmerspub"
}
Most important thing to remember is the PUT payload should have all the fields of the resource when updating or else the resource is overwritten with the data we send.
For example consider this PUT request where we send only the updated blog title.
HTTP PUT https://programmerspub.com/blog/general/5
Payload:
{
"blog_title": "What is PUT - updated",
"blog_post": "Confused whether to use PUT or PATCH or POST...updated post...",
"blog_post_author": "programmerspub"
}
After the PUT request only the blog title has the updated value while blog_post and blog_post_author fields are lost.
{
"blog_title": "What is PUT - updated"
}
PUT is idempotent and are not cacheable.
PATCH is another HTTP method which is used to update a resource with partial data. Unlike PUT, PATCH does not need the full payload to update a resource. For example if a resource has 100 fields, using PATCH would be a better option than PUT as PUT requires all 100 fields to be sent again to update a resource.
HTTP PATCH https://programmerspub.com/blog/general/5
Payload:
{
"blog_title": "What is PATCH - updated"
}
After the PATCH request, only the field blog_title is update while other fields remain intact.
{
"blog_title": "What is PATCH - updated",
"blog_post": "Confused whether to use PUT or PATCH or POST...updated post...",
"blog_post_author": "programmerspub"
}
PATCH is neither safe nor idempotent.
POST and PUT are both popular HTTP methods that are used interchangeably as they are both used to create and update a resource.
However, it is important to understand that POST should ideally be used to create a resource while PUT is to update a resource.
POST works on a collection while PUT works on a single resource.
So, while designning your API, understand the HTTP verbs idempotency and your use case to define the correct methods for the API calls.